Assembly Language Programming
Example Program
Flow Chart for Average Program
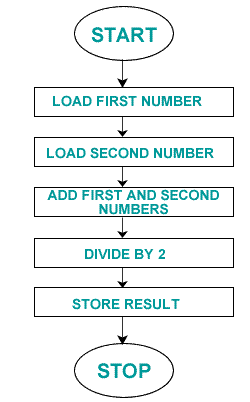
The task: get two numbers from distinct locations in memory. Add the numbers together and average the sum, store the average to a third location in memory.
The algorithm to implement this task can be described as a flow chart (see the accompanying diagram). Using the flow chart the algorithm can then be translated into an assembly language program as shown below.
Note the use of PC-relative addressing to load the addresses for the input and the output of the program into registers. This is followed by register-indirect addressing to move the actual input values into the working registers. The actual division is performed using an arithmetic shift right operation which returns an integer quotient. This result is then stored to memory using register-indirect addressing.
The remaining lines of assembly language code form a "wrapper" for the heart of the program. The AREA directive identifies that the program name is "Average" and that the succeeding instructions are read-only code. The ENTRY directive indicates the first executable instruction.
The SWI instruction causes the program to exit cleanly and return to the ARM debugger. The END directive marks the end of the file.
Average Program | |||
---|---|---|---|
AREA | Average, CODE, READONLY | ; name code block | |
ENTRY | ; marker of first executable instruction | ||
start | |||
ADR | r1, FIRSTNUM | ; load first address into r1 | |
ADR | r2, SECONDNUM | ; load second address into r2 | |
ADR | r3, AVGRESULT | ; load result address into r3 | |
LDR | r4, [r1] | ; load first number into r4 | |
LDR | r5, [r2] | ; load second number into r5 | |
ADD | r0, r4, r5 | ; add numbers together | |
MOV | r0, r0, ASR#1 | ; divide by two using arithmetic shift | |
STR | r0, [r3] | ; store result to address pointed to by r3 | |
SWI | 0x11 | ; terminate the program | |
END | ; marks the end of the file |